In this article I want to have a look at what is in store if you want a display for your embedded project.
Family picture
First, let me separate displays in 2 main families:
- Pixel matrix displays, on which you draw images pixel by pixel
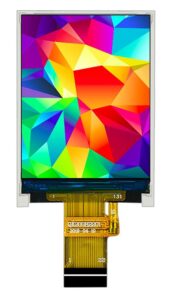
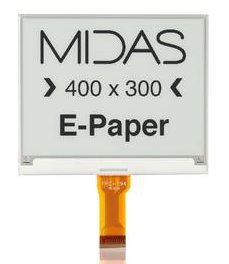
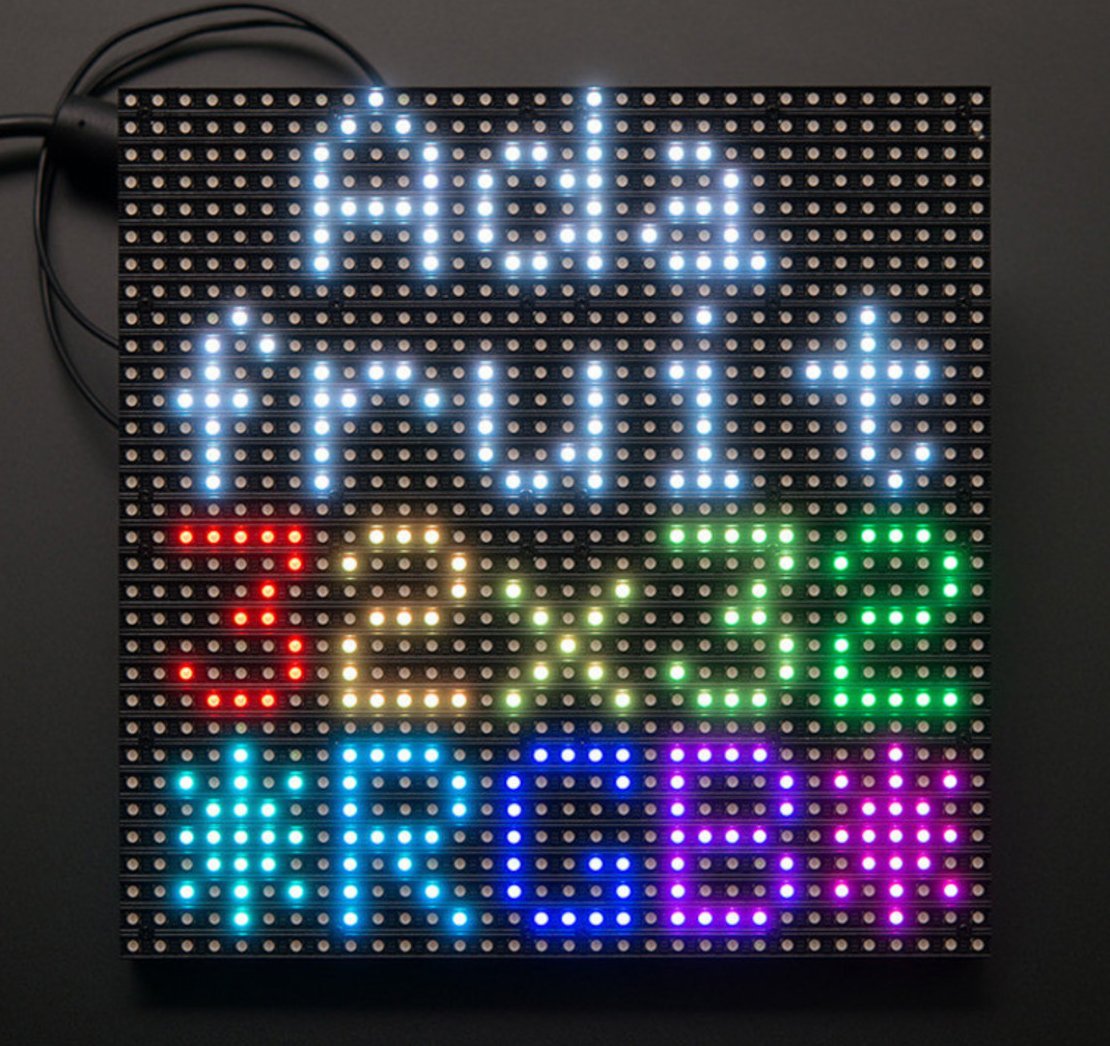
A TFT LCD display, an e-paper display, and a LED matrix display
- Segment displays, alphanumeric displays or other specialized displays on which you light-up segments or other pre-defined shapes. For instance, character LCD are modules on which you have a bunch of 5×8 pixel rectangles, they can display pre-defined letter.
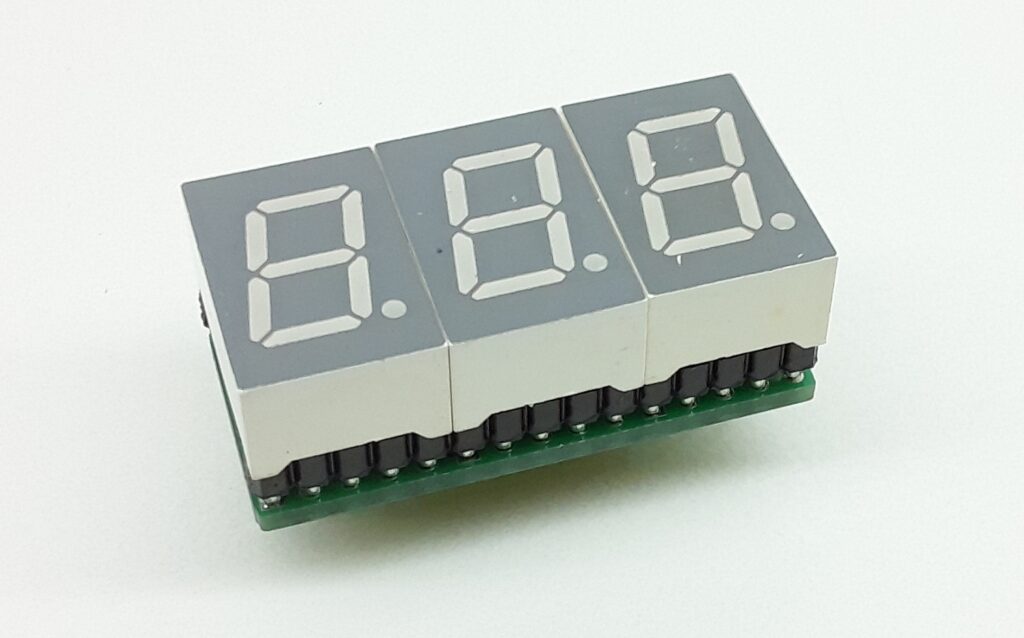
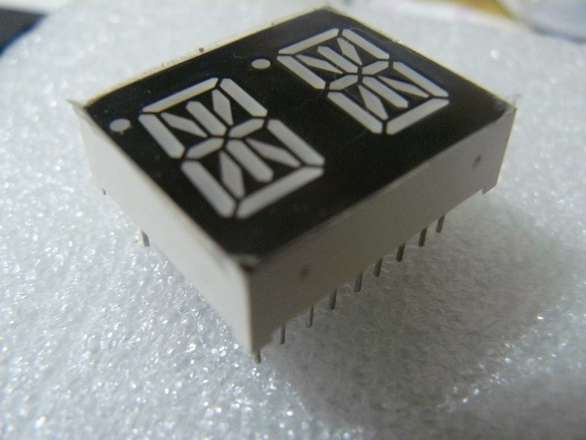
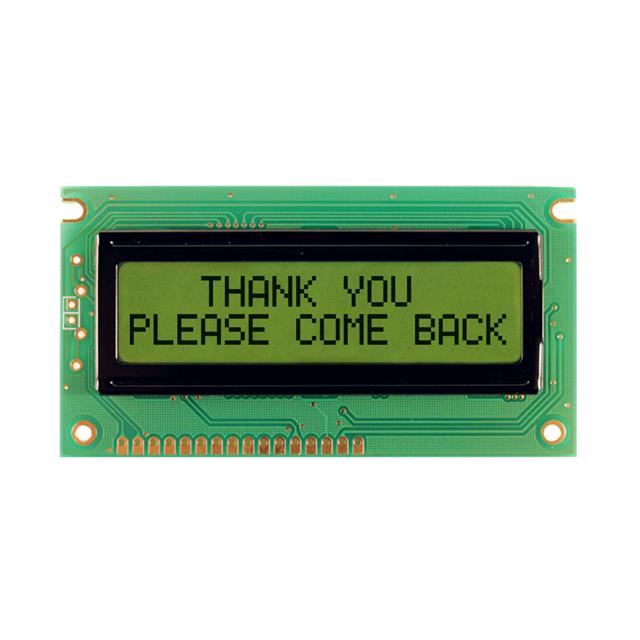
7-segment LED display, 14-segment LED display, and alphanumeric OLED display
For instance, in Digikey Optoelectronics section, displays are sorted like this:
- LCD, OLED, Graphic for matrix pixel
- LCD, OLED Character and Numeric for alphanumeric and specific OLED displays
- LED Character and Numeric for LED segment displays
In this article, I will only talk about pixel matrix displays.
Sourcing
Displays are usually sold as modules which contain a chip named display controller. The module also includes the display panel itself, mechanical elements such as frame, cable, connector, and sometimes backlighting or resistive touch panel.
Modules have lots of parameters that need to be tailored to applications: size of display, length of cable, mechanical assembly, communication protocol… it is difficult to sell one size fits all. Most companies will order custom modules from manufacturer directly.
However, for DIY and small batches, it is a more complicated situation. Where to buy then?
- Specialized DIY catalogues do the job of ordering display batches and resell details for you, with added documentation and support: Adafruit, Sparkfun, Seeed…
- You can also find some stocks in the usual distributors: Digikey, Farnell, LCSC with some disclaimers:
- Be mindful about stocks (LCSC stocks are usually one-shot, you might not find your reference restocked after they sold their stash)
- Dig everywhere. For some reason, Digikey has a good selection of e-ink displays when LCSC is better for OLED displays. And it changes, all the time.
- Scrapping!
- Best price, and saving the planet
- Display connectors are not easy to hack, a custom PCB could be the only way
- If you scrap a display, don’t forget that datasheets can be hard to come by, you might need to reverse-engineer for pinout and protocol.
- For instance this guy on Youtube bought a second-hand batch of supermarket e-labels (dubbed ESL: electronic shelf labels)
About OLED and e-inks: you will find mostly monochrome displays (not greyscale: monochrome, only black and white). The main difference is that e-ink retains image when powered off whereas OLED has to be refreshed, but OLED is faster, you cannot really display animations on e-inks.
Prices range
- 88×48 monochrome OLED: < 1 € on LCSC
- 320×240 color TFT LCD: around 5 € to 20 €*
- 152×296 e-ink display: around 4 €
* More expensive with resistive touchscreen
You can also find smart displays, which includes their embedded graphics layer, similar to what LVGL does, plus touchscreens, plus audio speakers, for instance the Riverdi EVE line. Of course it has its cost, it will be around 60 € for a 320×240 TFT LCD display.
Pixel physics
Just a quick note about how those display works.
OLED
In OLED displays you have matrix of tiny LEDs. It’s like a LED matrix display, but tiny.
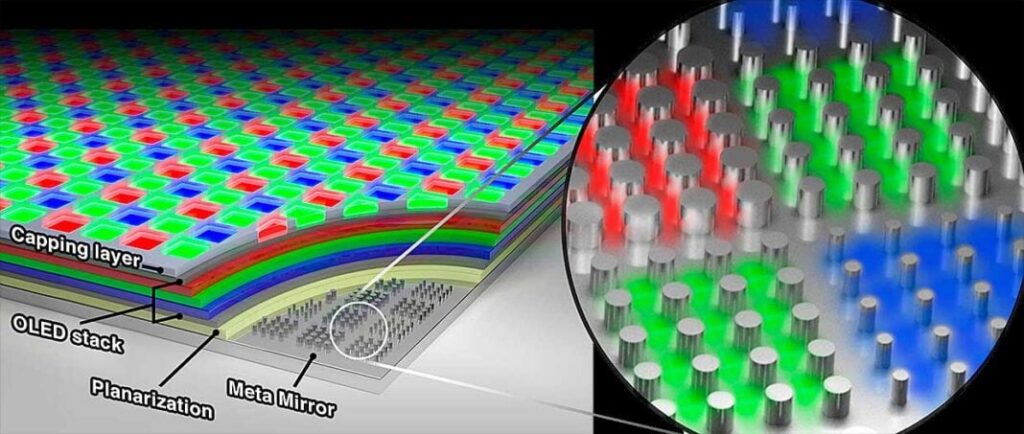
LCD
In LCD displays, you have a fixed RGB LED matrix, and you control a layers of a weird chemical thing called liquid crystals, thanks to an electric field. You can set this crystal gooey layer to be polarized at 90° with a fixed polarized layer, so they act like a shutter on your pixel color. Put them back at 0° and it lets everything through. And you can set any value in between!
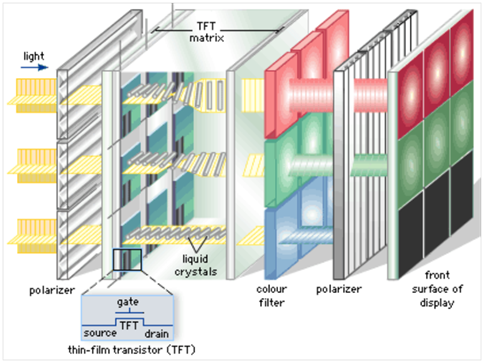
“TFT” refers to the transistors used to create the electric field.
E-ink
In e-ink displays each pixel is a bubble containing charged ink, black and white, they have different polarities and you use fields to set the ink at the top or at the bottom of the bubble. What’s cool is that it is flippy-floppy so each bubbles retains its state indefinitely. And also you don’t need backlighting, you can just use normal room light reflection to see the display – which is why it is called “e-paper”.
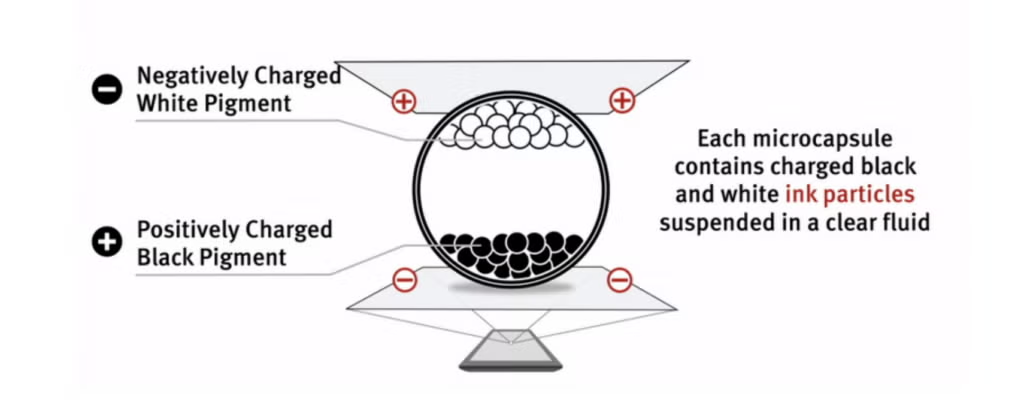
Display controllers
What are they?
A display controller is a bit like a huge GPIO expander, because you will never have enough GPIO on your nice standard microcontroller to drive a graphic display on your own.
In a TFT LCD display: each pixel color has its own transistor (TFT: Thin-Film-Transistor). It is disposed in a grid, so the number of GPIO lines needed is:
Number of row * Number of columns * Number of colors
Pixel control GPIO pile up quickly!
For instance, Ilitek ILI9341 is a controller able to drive a 320×240 TFT LCD display. It has 1040 GPIO pins to control the pixels, and there is a total 1278 pins on this chip.
- TFT Source Driver: 720 pins (240 x 3 RGB)
- TFT Gate Driver: 320 pins
From reading the datasheet, I understand it is supplied to the module manufacturer without any package, just a 16×0.7 micrometers piece of silicon.
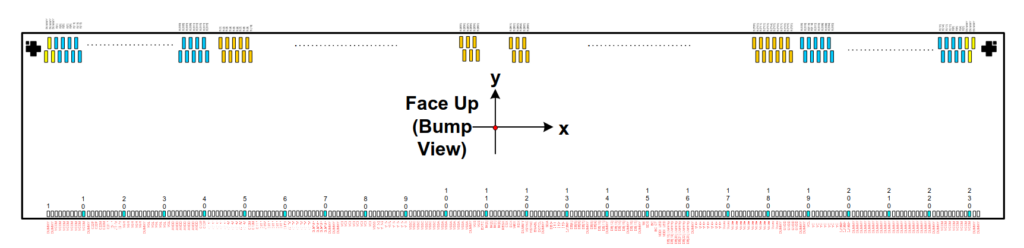
Mechanical pinout for the ILI9341 controller
Still lots of references
Since they are mostly custom-tailored, display modules are a chaotic mess of custom references with elusive and confidential datasheets. In comparison, display controllers are more standard components, most display modules will use a limited set of references.
However there are still quite a bunch of components in the market, because:
- Each different display tech needs different controllers
- A given controller handles a bunch of similar display sizes, e.g. 320×240 can also handle 240×320 and 240×240, but you will still need different size ranges
- Very similar products from different manufacturers tend to happen: in that case, they will have the same register commands, or very similar ones. It will not be mentioned in the datasheets, so googling said components will reveal their similarities.
Here is a non-exhaustive list of controllers that Zephyr RTOS supports:
- Sitronix ST77xx series for TFT LCD
- Ilitex ILI9xxx series or TFT LCD
- ST OTM8009a for TFT-LCD
- Galaxycore GC9x01x for TFT LCD
- Sharp LS0xx for TFT LCD
- Solomon SSD1306 for OLED/PLED
- Ultrachip UC81xx for dot-matrix ESL (Electronic Shelf Label, either e-paper or LCD)
- Analog Devices MAX7219 for 8-digits 7-segment LED display
Communication
Now, our main microcontroller needs to talk to the display module’s controller. Module controllers can even give you several different protocol options, in that case, they generally are set up via configuration resistors on your board.
SPI with DC – 4 wires protocol – MIPI DBI
Most module controllers use a slightly modified version of SPI. The main differences are:
- Adding a data/command GPIO to differentiate commands from pixel values, in order not to accidentally send a command when you request your favourite nuance of green.
- Removing the POCI (Peripheral Out, Controller In) or MISO or SDIN line. We talk, it listens.
- There is a Chip Select line, but you should probably not mux anything on this SPI lines because it will get quite busy with all those pixel values.
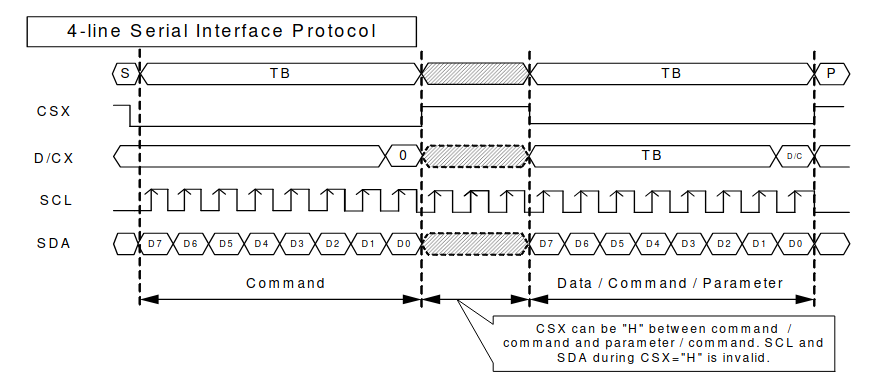
4-line protocol description from ILI9341 datasheet
Here you notice the name convention is the I2C one: SCL for clock and SDA for MOSI / SDOUT lines. But in the wild, any naming convention can happen.
I2C – 3 wires protocol
If you prefer invoking I2C peripherals, it is also possible. In this case:
- Chip Select and Data / Command are merged
- A bit has to be added to packets in order to mark data and command payloads
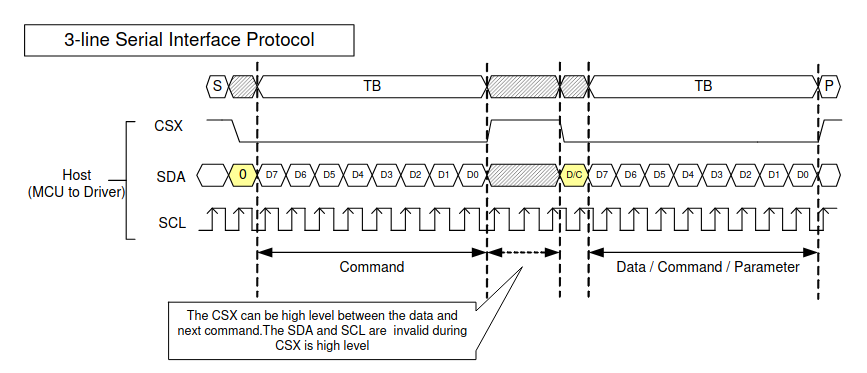
3-line protocol description from ILI9341 datasheet
Serial-parallel protocols
Same as before, but instead of sending data in a pure serial fashion, we add parallel lines, for instance here we have 8 additional cables in order to send a byte on one single clock front.
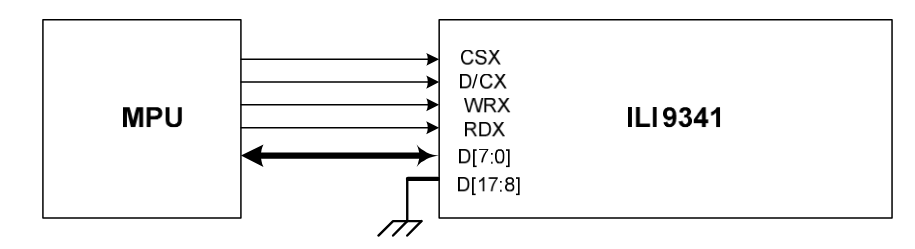
8080 serial connection from ILI9341 datasheet
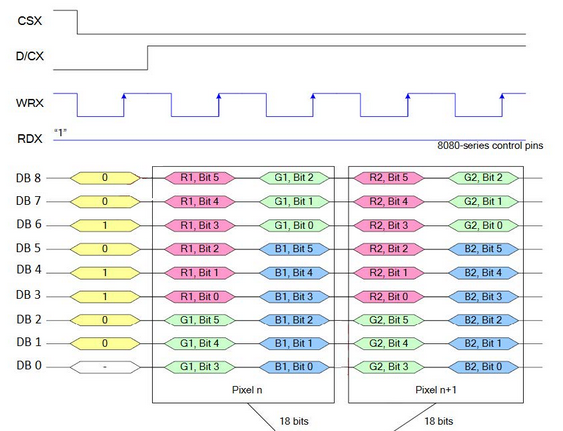
8080-I from this article
Note that those protocols are called 8080-I and 8080-II, because they were created for the Intel 8080 8-bit processor. So vintage!
The exception of Parallel RGB
In some particular cases, you can still buy a display without a controller, and use time multiplexing to reduce the number of GPIOs. This technique works with TFT LCD display, because if the refresh rate is fast enough, the capacitors can retain the pixel state long enough to wait for the next refresh, so it does not need one of those fancy display controllers with RAM.
There are no commands here, which means you cannot just update one pixel or play with RAM to create a swap effect. You just continuously feed all the RGB bytes at the rhythm of synchronization clocks.
I am not going to give more details on this interface here, because for your typical user GUI, SPI will be perfect. However, an interesting use case for parallel port is: making your own smart display module.
If you want to know more, read this article:
SoC support for serial RGB
You can buy SoCs with full parallel display interfaces for TFT LCD.
Manufacturer gave their different names, for additional fun:
- STM32 TFT-LCD (LTCD)
- Microchip MCUX eLCDIF
- Renesas LCDC
Display controller support for serial RGB
Some display controllers also have support for parallel RGB protocols on their side, to deliver the highest refresh rates.
In ILI9341 datasheet: “ILI9341 also supports the RGB interface for displaying a moving picture. When the RGB interface is selected, display operation is synchronized with external signals, VSYNC, HSYNC, and DOTCLK and input display data is written in synchronization with these signals according to the polarity of enable signal (DE)”.
Conclusion
The experience I have with embedded display in the companies I worked for is that it is a pain point: there are no standards, the manufacturers can only provide samples for some references, thus companies tend to stick to one display reference and copy they magic driver directory from one project to another. That can be solved thanks to Zephyr RTOS driver base, as I will explain in my next article Display software implementation using Zephyr RTOS.
More reading
- A cool article about embedded displays: https://predictabledesigns.com/introduction-embedded-electronic-displays/
- The ILI9341 datasheet is an interesting read, it has lots of information about communication protocols: https://cdn-shop.adafruit.com/datasheets/ILI9341.pdf